Documenting Components
For a component to be usable as an independent building block, not only by machines but also by humans, it needs to have its own documentation. The documentation for each component is displayed in the 'Overview' tab of the Workspace UI and the Scope UI.
#
Environment-specific templatesBit automates component documentation by parsing its code and displaying the output in a template provided by the environment used by that component.
Using different templates for different types of components (each using a different environment) means your components get documented in a way that makes sense for them. In addition to that, each documentation template provides a different API that uses the JS flavor or framework in use by the documented component. That means an Angular component will be documented using Angular and not, for instance, React.
#
Development vs ProductionDocumentation in development, for authored or modified components, will be shown in the Workspace UI. These docs will be generated using the 'DevServer' environment service to enable features needed for development, like "hot reloading".
The "production" version of the documentation, for component release versions, will be shown in the Scope UI and in the Workspace UI, for previous tag releases. The "production" version is generated using the 'Preview' service and provides an optimized built.
#
Customizing the documentationThe documentation can be customized in two ways:
Using the documentation template API for ad-hoc modifications. This is done to add custom components or to override a section in a specific component documentation. Learn about the React, React Native and Node environments API here.
Creating a new documentation template. This can be done as part of an environment extension or as part of a new environment aspect.
#
Create a documentation fileWhen using the bit create
command to create components, a docs.mdx
file will already be created for you.
To start customizing your documentation, create a documentation file in the component directory. Choose between a JSX or an MDX file (to learn about the MDX syntax, see here).
#
MDX doc filecomponent-name.docs.mdx
#
JS doc filecomponent-name.docs.tsx
#
Title / Display NameThe component's name.
Using MDX (front matter):
---displayName: Button---
Using JS:
export const title = 'my new customized title';
#
Abstract / DescriptionThe component description. To override, insert the following in the *.docs.*
file:
Using MDX (front matter):
---description: my customized title---
Using JS:
export const abstract = 'my new customized title';
#
LabelsKeywords that describe and categorize the component (used by Bit.dev's search engine).
To override, insert the following in the *.docs.*
file:
Using MDX: (front matter)
---labels: ['react', 'typescript', 'ui']---
Using JS:
export const labels = ['react', 'typescript', 'ui'];
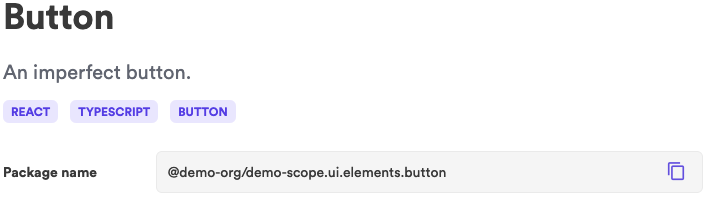
#
Custom sectionThe custom JSX slot gives you the freedom to extend the documentation page as you like.
Using MDX:
This can be done by simply writing down MDX in the component's *.docs.mdx
file, which can include simple markdown, as well as JSX.
Never import React to MDX doc files as it is injected by default.
import { Card } from './card';
### This is a custom section
Here's a React Card Component:
<Card />

Using JSX: Create a function with the name 'Overview' and export it as default:
export default function Overview() { return <h3>My custom docs section</h3>;}
#
Live examplesExamples are descriptions and playable code that instruct on how a component should be used.
To add live examples, insert the following in the *.docs.*
file:
Using MDX:
```jsx live=true () => { return <p>Hello!</p> } ```
Using JSX:
Create an examples
variable.
The examples
variable receives an array of objects, each representing a single example and each contains the following data (keys):
- scope: An object with all relevant imports.
- title: A string for the example title.
- Description: A string for the example description.
- Code: A string (template literal) for the example code.
Import the 'Card' component and set the examples
variable with a single object:
import React from 'react';import { Card } from './card';
export const examples = [ { scope: { Card }, title: 'Simple Card', description: "Use 'fullWidth' for small screens", code: `<Card size='fullWidth'> <p>When do two functions fight?</p> <p>- When they have arguments</p> </Card>` }];
#
Properties TableThe properties table is only available for the React and React Native environments
To ensure the documentation is faithful to the code, Bit generates the properties table from the code itself using react-docgen. At the bottom of the overview page you'll find all the component props listed and characterized in a table. These props are extracted from the JSDoc, prop-types and typescript type definitions, as well as the run-time code itself.
#
TypeScript + JSDocsexport interface IButton extends ButtonHTMLAttributes<HTMLButtonElement> { /** Choose between primary and secondary styling. */ variant?: 'primary' | 'secondary';}
export const Button = ({ children, variant, ...rest }: IButton) => { return ( <button className={styles[variant]} {...rest}> {children} </button> );};
Button.defaultProps = { variant: 'primary'};
A few things to note here:
- JSDocs comments written directly above the type definitions, will show up as prop description in the properties table.
- Inherited props, often received by extending React's out-of-the-box types, will not show up in the documentation unless they are explicitly defined. For example, in the code snippet above, a Button component extends a native HTML button attributes (
ButtonHTMLAttributes<HTMLButtonElement>
) but none of these attributes will appear in the props table (for example:disabled
,onclick
, etc.) - Conflicts between the different parts of the code that is parsed to the properties table, will be resolved one way or the other. So, make sure to keep all parts in coherence.
export interface IButton extends ButtonHTMLAttributes<HTMLButtonElement> { variant: 'primary' | 'secondary';}
export const Button = ({ children, variant = 'primary', ...rest }: IButton) => { return ( <button className={styles[variant]} {...rest}> {children} </button> );};
The above code shows 'variant' as a 'required' prop (since that is the default). However, the 'variant' prop receives a default value, making it 'optional'. The properties table will ignore TS and show the prop as 'optional'.
#
prop-types + JSDocsexport const Button = ({ children, variant, ...rest }) => { return ( <button className={styles[variant]} {...rest}> {children} </button> );};
Button.defaultProps = { variant: 'primary'};
Button.propTypes = { /** Choose between primary and secondary styling. */ variant: PropTypes.oneOf(['primary', 'secondary']), children: PropTypes.any.isRequired};
A few things to note here:
- JSDocs comments written directly above the prop type definitions, will show up as prop description in the properties table.
- Conflicts between the different parts of the code that is parsed to the properties table, will be resolved one way or the other. So, make sure to keep all parts in coherence.